Documentation / Manuel développeur
Modules disponibles
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Modeler
v4.10.0
|
#include <TANNModeler.h>
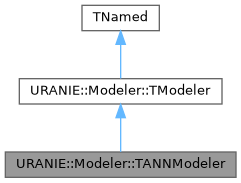
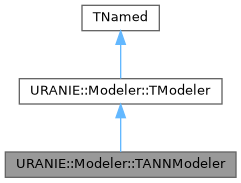
Public Types | |
enum | EProblem { kRegression, kClassification } |
enum | ENorm { kCR, kMinusOneOne, kZeroOne } |
Public Member Functions | |
Constructor and Destructor | |
TANNModeler (URANIE::DataServer::TDataServer *tds, TString architecture, Double_t dratio=0.80, Option_t *option="") | |
Constructor with a dataserver. More... | |
virtual | ~TANNModeler () |
Default destructor. More... | |
Setter and Getter function | |
Int_t | getNInput () |
Gets the number of input. More... | |
Int_t | getNHidden () |
Gets the number of hidden neurons. More... | |
Int_t | getNOutput () |
Gets the number of output. More... | |
Int_t | getNAPP () |
Gets the number of pure training patterns ("APPrentissage") More... | |
Int_t | getNTest () |
Gets the number of pure training patterns ("APPrentissage") More... | |
void | setWeights (ColumnVector weights) |
Sets the weigts. More... | |
ColumnVector | getWeights () |
Double_t | getWeight (Int_t ind) |
Returns the weight givent by the index. More... | |
Double_t | getMinValue (Int_t ind) |
Double_t | getMaxValue (Int_t ind) |
Double_t * | getdval () |
void | setValue (Int_t ind, Int_t &ncrt, Double_t *xy, Bool_t normalize=true) |
void | setDataSet (URANIE::DataServer::TPatternsEventList *tel) |
void | setMix (Bool_t bmix=kTRUE) |
void | setFcnTol (Double_t dprec) |
Double_t | getFcnTol () |
void | setNormalization (ENorm n) |
Sets the normalisation. More... | |
ENorm | getNormalization () |
Get the normalisation. More... | |
EProblem | getProblem () |
Get the problem type. More... | |
void | setSeed (Int_t ind=0) |
Init the seed. More... | |
Int_t | getSeed () |
Returns the seed value. More... | |
const char * | getInputName (Int_t i) |
Return the name of input attributes indexed by i. More... | |
const char * | getOutputName () |
Get the attribute name of the output. More... | |
Training | |
void | init () |
void | randomizeWeights () |
randomize the weights More... | |
void | train (Int_t niter=10, Int_t nInit=10, Option_t *option="text", Bool_t useGPU=false) |
Double_t | getEQM (Option_t *option="") |
Gets the EQM. More... | |
Double_t | getWeightDecay () |
Get the weight decay parameter. More... | |
void | saveIfBetter (const ColumnVector &weights, Double_t eqm_app, Double_t eqm_tst) |
Save a vector of weight if the RMS on the test database is better than what we currently have. More... | |
Exports function | |
The exports function to save an Artificial Neural Networks in an external format
The user fonction is the exportFunction method. tann->exportFunction("c++", "uranie_ann_flowrate.C","ANNflowrate"); tann->exportFunction("fortran", "uranie_ann_flowrate.f","ANNflowrate"); tann->exportFunction("pmml", sPMMLFile, sANNName,"new");
tann->exportFunction("C++,fortran,pmml", "uranie_ann_flowrate","ANNflowrate"); | |
void | exportModelCplusplus (const char *file="", const char *name="", Option_t *option="") const |
The only user interface method. More... | |
void | exportModelCplusplus (std::ofstream *sourcefile) const |
void | exportModelPMML (const char *file="", const char *name="", Option_t *option="") const |
Export the TANNModeler object in a PMML File. More... | |
void | exportModelFortran (const char *file, const char *name, Option_t *option="") |
Export the ANN model in a Fortran file. More... | |
void | exportModelFortran (std::ofstream *sourcefile) const |
void | exportModelPython (std::ofstream *sourcefile) const |
Export the model in Python langage in a file (not yet implemented) More... | |
Printing Log | |
virtual void | printLog (Option_t *option="") |
![]() | |
TModeler (URANIE::DataServer::TDataServer *tds, TString architecture, Option_t *option="") | |
Constructor with a dataserver. More... | |
TModeler (URANIE::DataServer::TDataServer *tds, const char *varexpinput, const char *varexpoutput, Option_t *option="") | |
Default constructor with input and output attributes. More... | |
virtual | ~TModeler () |
Default destructor. More... | |
TVectorD | getParameters () |
Return the parameter vector of the model. More... | |
Double_t | getR2 () const |
Return the R2 quality. More... | |
const char * | getInputName (Int_t i) const |
Return the name of input attributes. More... | |
const char * | getOutputName (Int_t i=0) |
Return the name of output attribute. More... | |
Int_t | getNInput () |
Gets the number of input. More... | |
Bool_t | getIntercept () |
Gets the boolean attribut _bIntercept. More... | |
void | setDrawProgressBar (Bool_t bbool=kTRUE) |
Set the "draw progress bar" flag. More... | |
Bool_t | getDrawProgressBar () |
Get the "draw progress bar" flag. More... | |
void | train (Option_t *option="text") |
virtual void | exportFunction (const char *lang, const char *file="", const char *name="", Option_t *option="") |
Export the model in an external file with a specified langage. More... | |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
Public Attributes | |
Bool_t | _blog |
Boolean for edit the log. More... | |
URANIE::DataServer::TDataServer * | _tds |
UMLP * | _UMLP |
Pointeur vers un TDS. More... | |
![]() | |
Bool_t | _blog |
Boolean for edit the log. More... | |
Bool_t | _bdrawProgressBar |
Boolean to know if the progress bar has to be drawn. More... | |
Bool_t | _bStoreYHat |
Boolean to specify if we add the {} attribute in the TDS [default kTRUE]. More... | |
URANIE::DataServer::TDataServer * | _tds |
Int_t | _nS |
Pointer to a TDS. More... | |
TString | _sInputAttributes |
The string of input attributes (separated by the character ":". More... | |
TString | _sOutputAttributes |
The string of output attributes (separated by the character ":". More... | |
TString | _sIntermediateArchitecture |
The intermediate (between the first and last character ",") information. More... | |
TList * | _listOfInputAttributes |
The list of input Leaf. More... | |
TList * | _listOfOutputAttributes |
The list of output Leaf. More... | |
Int_t | _nX |
The number of input attributes. More... | |
Int_t | _nY |
The number of output attributes. More... | |
TString | _sFileName |
The name of the file when export the model without extension. More... | |
TString | _sFunctionName |
The name of the function when export the model. More... | |
TVectorD | _parameterValues |
The list of parameters. More... | |
Double_t | _dr2 |
The R2 quality. More... | |
Bool_t | _bIntercept |
For certain models, add the intercept (Default is TRUE) More... | |
Protected Attributes | |
Int_t | _nSeed |
Private Attributes | |
Int_t | _nInput |
! Seed for the random weight initialization More... | |
Int_t | _nHidden |
! The number of inputs More... | |
Int_t | _nHiddenLayer |
! The number of hidden neurons More... | |
Int_t | _nOutput |
! the number of hidden layer More... | |
Int_t | _nNeurons |
! The number of outputs More... | |
Int_t | _nApp |
! The number of neurons More... | |
Int_t | _nTest |
! Number of samples in the training set dedicated to back-propagation More... | |
Double_t | _dLearn |
! Number of samples in the training set dedicated to validation More... | |
Double_t | _dTest |
! The learning quality More... | |
Double_t | _dWeightDecay |
! The test quality More... | |
URANIE::DataServer::TPatternsEventList * | _elAll |
! The weight decay parameter - when used, favorize a smoother ANN More... | |
Bool_t | _bMix |
! The mix list of patterns More... | |
TString | _sArchi |
! True if data set must be mixed More... | |
TString | _sInput |
! The architecture, e.g. "x:y:z,3,yhat" specifying inputs, number of hidden neurons, and output More... | |
TString | _sHidden |
TString | _sOutput |
Int_t | _nWeight |
ColumnVector | _vecWeights |
! The number of weights More... | |
TempSolution * | _tmpSolution |
ColumnVector | _vecMinValues |
ColumnVector | _vecMaxValues |
! Min values, used for normalization More... | |
TList * | _listOfAttributes |
! Max values, used for normalization More... | |
Parameters of algorithm | |
Double_t | _dFcnTol |
ENorm | _nNormType |
! The function tolerance for the trust region algorithm More... | |
EProblem | _nProlemType |
! The normalized for input and output More... | |
Double_t * | _dval |
! The type of problem (Regression, Classification) More... | |
Static Private Attributes | |
static const int | OPTIM_MAX_ITER = 10000 |
static const int | OPTIM_MAX_FEVAL = 50000 |
Member Enumeration Documentation
◆ ENorm
◆ EProblem
Constructor & Destructor Documentation
◆ TANNModeler()
URANIE::Modeler::TANNModeler::TANNModeler | ( | URANIE::DataServer::TDataServer * | tds, |
TString | architecture, | ||
Double_t | dratio = 0.80 , |
||
Option_t * | option = "" |
||
) |
Constructor with a dataserver.
◆ ~TANNModeler()
|
virtual |
Default destructor.
Member Function Documentation
◆ exportModelCplusplus() [1/2]
void URANIE::Modeler::TANNModeler::exportModelCplusplus | ( | const char * | file = "" , |
const char * | name = "" , |
||
Option_t * | option = "" |
||
) | const |
The only user interface method.
- Parameters
-
lang (const char*) the keyword for the external format [c++|fortran|pmml] file (const char*) the filename. If empty, it is the name of the TDS with the good extension.[""] name (const char*) name of the function. option (const char*) option parameter. - NEW : create a new PMML file
- UPDATE : Write the current ANN in the PMML file and if already exist an ANN in the PMML file, erase it.
- BACKUP : Write the current ANN in the PMML file and if already exist ANNs in the PMML file with modelname "name_I", rename the old with "name_I+1"Export the ANN model in a ROOT macro file
◆ exportModelCplusplus() [2/2]
|
virtual |
Implements URANIE::Modeler::TModeler.
◆ exportModelFortran() [1/2]
void URANIE::Modeler::TANNModeler::exportModelFortran | ( | const char * | file, |
const char * | name, | ||
Option_t * | option = "" |
||
) |
Export the ANN model in a Fortran file.
◆ exportModelFortran() [2/2]
|
virtual |
Implements URANIE::Modeler::TModeler.
◆ exportModelPMML()
|
virtual |
Export the TANNModeler object in a PMML File.
Implements URANIE::Modeler::TModeler.
◆ exportModelPython()
|
inlinevirtual |
Export the model in Python langage in a file (not yet implemented)
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
◆ getdval()
|
inline |
Referenced by ClassImp().
◆ getEQM()
Double_t URANIE::Modeler::TANNModeler::getEQM | ( | Option_t * | option = "" | ) |
Gets the EQM.
- Parameters
-
option ["learn|test"] give the Mean Square Error (MSE ) on the learn database ("learn") or on the test database ("test").
◆ getFcnTol()
|
inline |
◆ getInputName()
|
inline |
Return the name of input attributes indexed by i.
◆ getMaxValue()
|
inline |
Referenced by ClassImp().
◆ getMinValue()
|
inline |
Referenced by ClassImp().
◆ getNAPP()
|
inline |
Gets the number of pure training patterns ("APPrentissage")
Referenced by ClassImp().
◆ getNHidden()
|
inline |
Gets the number of hidden neurons.
Referenced by ClassImp().
◆ getNInput()
|
inline |
Gets the number of input.
Referenced by ClassImp().
◆ getNormalization()
|
inline |
Get the normalisation.
Referenced by ClassImp().
◆ getNOutput()
|
inline |
Gets the number of output.
Referenced by ClassImp().
◆ getNTest()
|
inline |
Gets the number of pure training patterns ("APPrentissage")
Referenced by ClassImp().
◆ getOutputName()
|
inline |
Get the attribute name of the output.
◆ getProblem()
|
inline |
Get the problem type.
Referenced by ClassImp().
◆ getSeed()
|
inline |
Returns the seed value.
◆ getWeight()
|
inline |
Returns the weight givent by the index.
the index begins to 0 and ends to nW-1
◆ getWeightDecay()
|
inline |
Get the weight decay parameter.
Referenced by ClassImp().
◆ getWeights()
|
inline |
Referenced by ClassImp().
◆ init()
void URANIE::Modeler::TANNModeler::init | ( | ) |
◆ printLog()
|
virtual |
Reimplemented from URANIE::Modeler::TModeler.
◆ randomizeWeights()
void URANIE::Modeler::TANNModeler::randomizeWeights | ( | ) |
randomize the weights
◆ saveIfBetter()
void URANIE::Modeler::TANNModeler::saveIfBetter | ( | const ColumnVector & | weights, |
Double_t | eqm_app, | ||
Double_t | eqm_tst | ||
) |
Save a vector of weight if the RMS on the test database is better than what we currently have.
Referenced by ClassImp().
◆ setDataSet()
|
inline |
◆ setFcnTol()
|
inline |
◆ setMix()
|
inline |
◆ setNormalization()
void URANIE::Modeler::TANNModeler::setNormalization | ( | ENorm | n | ) |
Sets the normalisation.
◆ setSeed()
void URANIE::Modeler::TANNModeler::setSeed | ( | Int_t | ind = 0 | ) |
Init the seed.
If the value is zero, the seed is initialized with the hour in milliseconds
- Parameters
-
ind (Int_t) the seed value
◆ setValue()
void URANIE::Modeler::TANNModeler::setValue | ( | Int_t | ind, |
Int_t & | ncrt, | ||
Double_t * | xy, | ||
Bool_t | normalize = true |
||
) |
Referenced by ClassImp().
◆ setWeights()
|
inline |
Sets the weigts.
◆ train()
void URANIE::Modeler::TANNModeler::train | ( | Int_t | niter = 10 , |
Int_t | nInit = 10 , |
||
Option_t * | option = "text" , |
||
Bool_t | useGPU = false |
||
) |
Launch the training of the ANN. This potentially uses the GPU if available.
- Parameters
-
niter number of times (a random permutation of) the test base will be presented for training nInit number of times the ANN is trained (from random start weights) with a given permutation of the test database
Member Data Documentation
◆ _blog
Bool_t URANIE::Modeler::TANNModeler::_blog |
Boolean for edit the log.
◆ _bMix
|
private |
! The mix list of patterns
◆ _dFcnTol
|
private |
◆ _dLearn
|
private |
! Number of samples in the training set dedicated to validation
◆ _dTest
|
private |
! The learning quality
◆ _dval
|
private |
! The type of problem (Regression, Classification)
Temporary array used during training
◆ _dWeightDecay
|
private |
! The test quality
◆ _elAll
|
private |
! The weight decay parameter - when used, favorize a smoother ANN
◆ _listOfAttributes
|
private |
! Max values, used for normalization
◆ _nApp
|
private |
! The number of neurons
◆ _nHidden
|
private |
! The number of inputs
◆ _nHiddenLayer
|
private |
! The number of hidden neurons
◆ _nInput
|
private |
! Seed for the random weight initialization
◆ _nNeurons
|
private |
! The number of outputs
◆ _nNormType
|
private |
! The function tolerance for the trust region algorithm
◆ _nOutput
|
private |
! the number of hidden layer
◆ _nProlemType
|
private |
! The normalized for input and output
◆ _nSeed
|
protected |
◆ _nTest
|
private |
! Number of samples in the training set dedicated to back-propagation
◆ _nWeight
|
private |
◆ _sArchi
|
private |
! True if data set must be mixed
◆ _sHidden
|
private |
◆ _sInput
|
private |
! The architecture, e.g. "x:y:z,3,yhat" specifying inputs, number of hidden neurons, and output
◆ _sOutput
|
private |
◆ _tds
URANIE::DataServer::TDataServer* URANIE::Modeler::TANNModeler::_tds |
◆ _tmpSolution
|
private |
◆ _UMLP
UMLP* URANIE::Modeler::TANNModeler::_UMLP |
Pointeur vers un TDS.
Referenced by ClassImp().
◆ _vecMaxValues
|
private |
! Min values, used for normalization
◆ _vecMinValues
|
private |
◆ _vecWeights
|
private |
! The number of weights
! The vector of weights, stored like this: [a][b(1) b(2) b(3).... b(_nInput+2)][c(1) c(2) c(3).... c(_nInput+2)][ ... ] a: scalar value giving the weight of the output biais (link betw. single output and biais neuron in hidden layer) b, c, d, ...: _nHidden blocks of (_nInput+2) scalars representing: b(1): weight link between second hidden neuron and output (c(1) for the 3rd hidden neuron, d(1) for the 4th, etc ...) b(2): weight between biais input neuron and second hidden neuron b(2+i): weight between i-th (real) input neuron and second hidden neuron See impl. of UMPL::evaluateInput().
◆ OPTIM_MAX_FEVAL
|
staticprivate |
◆ OPTIM_MAX_ITER
|
staticprivate |