Documentation / Developer's manual
Available modules
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Modeler v4.9.0
|
#include <TGLM.h>
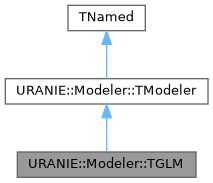
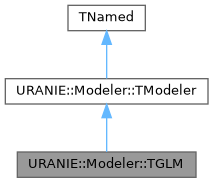
Public Types | |
enum | EDistribution { kUnknown , kPoisson , kGamma , kBinomial , kNormal } |
enum | ELinkFunctin { kCanonical , kIdentity , kLogit , kProbit , kLogLog , kLog , kInverse } |
Public Member Functions | |
Constructor and Destructor | |
TGLM (URANIE::DataServer::TDataServer *tds, TString architecture, Option_t *option="") | |
Default constructor with the TDataServer and the architecture. | |
TGLM (URANIE::DataServer::TDataServer *tds, const char *varexpinput, const char *varexpoutput, Option_t *option="") | |
Default constructor with input and output attributes. | |
virtual | ~TGLM () |
Default destructor. | |
Get and Set methods | |
void | setFamily (EDistribution nYDistribution=kUnknown, ELinkFunctin nLinkFunction=kCanonical) |
Set the family of error (Distribution of ![]() | |
EDistribution | getYDistribution () |
Return the type of Y distribution. | |
ELinkFunctin | getLinkFunction () |
Return the link function. | |
void | setMaxIteration (Int_t n) |
Set the number of maximum iteration. | |
Int_t | getNIteration () |
Get the number iterations at convergence. | |
Int_t | getMaxIteration () |
Get the number of maximum iteration. | |
void | setTolerance (Double_t dtol) |
Set the Tolerance. | |
Double_t | getTolerance () |
Get the Tolerance. | |
Double_t | getNullDeviance () |
Get the Null Deviance value. | |
Double_t | getResidualDeviance () |
Get the Residual Deviance value. | |
void | setInitialBeta (TVectorD vecIni) |
Set the initial parameter. | |
TVectorD | getInitialBeta () |
Get the initial parameter. | |
Estimate | |
void | estimate (Option_t *option="") |
void | fillData (TMatrixD &Aw, TVectorD &yw, TMatrixD &matY) |
Fill the data in a TMatrix and a TVector. | |
Export the model in extrenal langage | |
void | exportModelCplusplus (std::ofstream *sourcefile) const |
Export the model in C++ langage in a file. | |
void | exportModelFortran (std::ofstream *sourcefile) const |
Export the model in Fortran langage in a file. | |
void | exportModelPMML (const char *file="", const char *name="", Option_t *option="") const |
Export the model in a PMML file (not yet implemented) | |
void | exportModelPython (std::ofstream *sourcefile) const |
Export the model in Python langage in a file (not yet implemented) | |
Printing Log | |
void | printLog (Option_t *option="") |
![]() | |
TModeler (URANIE::DataServer::TDataServer *tds, TString architecture, Option_t *option="") | |
Constructor with a dataserver. | |
TModeler (URANIE::DataServer::TDataServer *tds, const char *varexpinput, const char *varexpoutput, Option_t *option="") | |
Default constructor with input and output attributes. | |
virtual | ~TModeler () |
Default destructor. | |
TVectorD | getParameters () |
Return the parameter vector of the model. | |
Double_t | getR2 () const |
Return the R2 quality. | |
const char * | getInputName (Int_t i) const |
Return the name of input attributes. | |
const char * | getOutputName (Int_t i=0) |
Return the name of output attribute. | |
Int_t | getNInput () |
Gets the number of input. | |
Bool_t | getIntercept () |
Gets the boolean attribut _bIntercept. | |
void | setDrawProgressBar (Bool_t bbool=kTRUE) |
Set the "draw progress bar" flag. | |
Bool_t | getDrawProgressBar () |
Get the "draw progress bar" flag. | |
void | train (Option_t *option="text") |
virtual void | exportFunction (const char *lang, const char *file="", const char *name="", Option_t *option="") |
Export the model in an external file with a specified langage. | |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
Private Attributes | |
EDistribution | _nDistribution |
The type of Y distribution. | |
ELinkFunctin | _nLinkFunction |
The link function (default kCanonical) | |
Int_t | _nIteration |
the number of iteration at convergence (default 0) | |
Int_t | _nMaxIteration |
the number of maximum iteration (default 25) | |
TVectorD | _vecBetaInit |
Inital Vector for beta. | |
Double_t | _dTolerance |
The tolerance to stop algorithm (default 1.e-6) | |
Double_t | _dNullDeviance |
The Null Deviance value. | |
Double_t | _dResidualDeviance |
The Residual Deviance value. | |
Additional Inherited Members | |
![]() | |
Bool_t | _blog |
Boolean for edit the log. | |
Bool_t | _bdrawProgressBar |
Boolean to know if the progress bar has to be drawn. | |
Bool_t | _bStoreYHat |
Boolean to specify if we add the \hat{} attribute in the TDS [default kTRUE]. | |
URANIE::DataServer::TDataServer * | _tds |
Int_t | _nS |
Pointer to a TDS. | |
TString | _sInputAttributes |
The string of input attributes (separated by the character ":". | |
TString | _sOutputAttributes |
The string of output attributes (separated by the character ":". | |
TString | _sIntermediateArchitecture |
The intermediate (between the first and last character ",") information. | |
TList * | _listOfInputAttributes |
The list of input Leaf. | |
TList * | _listOfOutputAttributes |
The list of output Leaf. | |
Int_t | _nX |
The number of input attributes. | |
Int_t | _nY |
The number of output attributes. | |
TString | _sFileName |
The name of the file when export the model without extension. | |
TString | _sFunctionName |
The name of the function when export the model. | |
TVectorD | _parameterValues |
The list of parameters. | |
Double_t | _dr2 |
The R2 quality. | |
Bool_t | _bIntercept |
For certain models, add the intercept (Default is TRUE) | |
Member Enumeration Documentation
◆ EDistribution
◆ ELinkFunctin
Constructor & Destructor Documentation
◆ TGLM() [1/2]
URANIE::Modeler::TGLM::TGLM | ( | URANIE::DataServer::TDataServer * | tds, |
TString | architecture, | ||
Option_t * | option = "" |
||
) |
Default constructor with the TDataServer and the architecture.
Referenced by ClassImp().
◆ TGLM() [2/2]
URANIE::Modeler::TGLM::TGLM | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | varexpinput, | ||
const char * | varexpoutput, | ||
Option_t * | option = "" |
||
) |
Default constructor with input and output attributes.
◆ ~TGLM()
|
virtual |
Default destructor.
Referenced by ClassImp().
Member Function Documentation
◆ estimate()
void URANIE::Modeler::TGLM::estimate | ( | Option_t * | option = "" | ) |
Referenced by ClassImp().
◆ exportModelCplusplus()
|
virtual |
Export the model in C++ langage in a file.
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ exportModelFortran()
|
virtual |
Export the model in Fortran langage in a file.
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ exportModelPMML()
|
inlinevirtual |
Export the model in a PMML file (not yet implemented)
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
◆ exportModelPython()
|
inlinevirtual |
Export the model in Python langage in a file (not yet implemented)
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
◆ fillData()
void URANIE::Modeler::TGLM::fillData | ( | TMatrixD & | Aw, |
TVectorD & | yw, | ||
TMatrixD & | matY | ||
) |
Fill the data in a TMatrix and a TVector.
- Todo:
- To deplace in the TModeler Class because it is the same for TLinearRegression.
Referenced by ClassImp().
◆ getInitialBeta()
|
inline |
Get the initial parameter.
References _vecBetaInit.
◆ getLinkFunction()
|
inline |
Return the link function.
References _nLinkFunction.
◆ getMaxIteration()
|
inline |
Get the number of maximum iteration.
References _nMaxIteration.
◆ getNIteration()
|
inline |
Get the number iterations at convergence.
References _nIteration.
◆ getNullDeviance()
|
inline |
Get the Null Deviance value.
References _dNullDeviance.
◆ getResidualDeviance()
|
inline |
Get the Residual Deviance value.
References _dResidualDeviance.
◆ getTolerance()
|
inline |
Get the Tolerance.
References _dTolerance.
◆ getYDistribution()
|
inline |
Return the type of Y distribution.
References _nDistribution.
◆ printLog()
|
virtual |
Reimplemented from URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ setFamily()
void URANIE::Modeler::TGLM::setFamily | ( | EDistribution | nYDistribution = kUnknown , |
ELinkFunctin | nLinkFunction = kCanonical |
||
) |
Set the family of error (Distribution of
- Parameters
-
nYDistribution (EDistribution) The distribution of (kBinomial|kPoisson|kGamma)nLinkFunction (ELinkFunctin) The link function. By defulalt, the canonical function, which depend of the Y distribution, is used. [kCanonical](kCanonical|kIdentity|kLogit|kProbit|kLog)
Referenced by ClassImp().
◆ setInitialBeta()
void URANIE::Modeler::TGLM::setInitialBeta | ( | TVectorD | vecIni | ) |
Set the initial parameter.
Referenced by ClassImp().
◆ setMaxIteration()
|
inline |
Set the number of maximum iteration.
References _nMaxIteration.
◆ setTolerance()
|
inline |
Set the Tolerance.
References _dTolerance.
Member Data Documentation
◆ _dNullDeviance
|
private |
The Null Deviance value.
Referenced by ClassImp(), and getNullDeviance().
◆ _dResidualDeviance
|
private |
The Residual Deviance value.
Referenced by ClassImp(), and getResidualDeviance().
◆ _dTolerance
|
private |
The tolerance to stop algorithm (default 1.e-6)
Referenced by ClassImp(), getTolerance(), and setTolerance().
◆ _nDistribution
|
private |
The type of Y distribution.
Referenced by ClassImp(), and getYDistribution().
◆ _nIteration
|
private |
the number of iteration at convergence (default 0)
Referenced by ClassImp(), and getNIteration().
◆ _nLinkFunction
|
private |
The link function (default kCanonical)
Referenced by ClassImp(), and getLinkFunction().
◆ _nMaxIteration
|
private |
the number of maximum iteration (default 25)
Referenced by ClassImp(), getMaxIteration(), and setMaxIteration().
◆ _vecBetaInit
|
private |
Inital Vector for beta.
Referenced by ClassImp(), and getInitialBeta().