Documentation / Developer's manual
Available modules
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Modeler v4.9.0
|
Description of the class TKernel. More...
#include <TKernel.h>
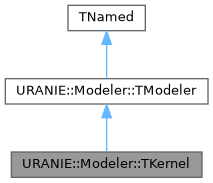
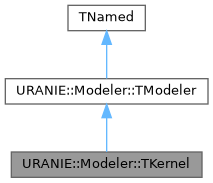
Public Types | |
enum | EKernelType { kUnknown , kLinear , kPoly , kSigmoid , kRBF , kLaplace , kSpline1 , kBernoulli1 } |
Public Member Functions | |
Constructor and Destructor | |
TKernel (URANIE::DataServer::TDataServer *tds, TString architecture, Option_t *option="") | |
constructor with the TDataServer and the architecture | |
TKernel (URANIE::DataServer::TDataServer *tds, const char *varexpinput, const char *varexpoutput, Option_t *option="") | |
Default constructor with input and output attributes. | |
virtual | ~TKernel () |
Default destructor. | |
Get and Set methods | |
void | setKernel (EKernelType nKernel) |
Set the type of kernel without parameter. | |
void | setKernel (EKernelType nKernel, Double_t dparam1) |
void | setKernel (EKernelType nKernel, Double_t dparam1, Double_t dparam2) |
Set the type of kernel with two parameters. | |
void | setKernel (EKernelType nKernel, Double_t dparam1, Double_t dparam2, Double_t dparam3) |
Set the type of kernel with three parameters. | |
EKernelType | getKernel () |
Return the type of Y distribution. | |
Double_t | getParameter1 () |
Return First Parameter. | |
Double_t | getParameter2 () |
Return Second Parameter. | |
void | setLambda (Double_t dlambda) |
Set the Lambda parameter. | |
Double_t | getLambda () |
Get the Lambda. | |
TMatrixDSym | getMatrixK () |
Get the matrix _matK. | |
Estimate | |
void | estimate (Option_t *option="") |
estimate | |
void | fillData () |
Fill the matrix of Inputs attributes and the vector of output attribute (nY=1) | |
void | computeK (Option_t *option="") |
compute the matrix K | |
Export the model in extrenal langage | |
void | exportModelCplusplus (std::ofstream *sourcefile) const |
Export the model in C++ langage in a file. | |
void | exportModelFortran (std::ofstream *sourcefile) const |
Export the model in Fortran langage in a file. | |
void | exportModelPMML (const char *file="", const char *name="", Option_t *option="") const |
Export the model in a PMML file (not yet implemented) | |
void | exportModelPython (std::ofstream *sourcefile) const |
Export the model in Python langage in a file (not yet implemented) | |
Printing Log | |
void | printLog (Option_t *option="") |
![]() | |
TModeler (URANIE::DataServer::TDataServer *tds, TString architecture, Option_t *option="") | |
Constructor with a dataserver. | |
TModeler (URANIE::DataServer::TDataServer *tds, const char *varexpinput, const char *varexpoutput, Option_t *option="") | |
Default constructor with input and output attributes. | |
virtual | ~TModeler () |
Default destructor. | |
TVectorD | getParameters () |
Return the parameter vector of the model. | |
Double_t | getR2 () const |
Return the R2 quality. | |
const char * | getInputName (Int_t i) const |
Return the name of input attributes. | |
const char * | getOutputName (Int_t i=0) |
Return the name of output attribute. | |
Int_t | getNInput () |
Gets the number of input. | |
Bool_t | getIntercept () |
Gets the boolean attribut _bIntercept. | |
void | setDrawProgressBar (Bool_t bbool=kTRUE) |
Set the "draw progress bar" flag. | |
Bool_t | getDrawProgressBar () |
Get the "draw progress bar" flag. | |
void | train (Option_t *option="text") |
virtual void | exportFunction (const char *lang, const char *file="", const char *name="", Option_t *option="") |
Export the model in an external file with a specified langage. | |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
Private Attributes | |
Private and Public attributes | |
EKernelType | _nKernelType |
The type of Kernel. | |
Double_t | _dParameter1 |
The First Parameter (gamma) [1.0]. | |
Double_t | _dParameter2 |
The Second Parameter (coeff) [0.0]. | |
Double_t | _dParameter3 |
The Third Parameter (degree) [1.0]. | |
Int_t | _nParameter |
The number of parameter. | |
TMatrixD | _matX |
The matrix of inputs attributes. | |
TVectorD | _vecY |
The vector of Y (outputs) | |
TMatrixDSym | _matK |
The matrix of K (inputs) | |
Double_t | _dLambda |
The lambda parameter (default 1.e-6) | |
Additional Inherited Members | |
![]() | |
Bool_t | _blog |
Boolean for edit the log. | |
Bool_t | _bdrawProgressBar |
Boolean to know if the progress bar has to be drawn. | |
Bool_t | _bStoreYHat |
Boolean to specify if we add the \hat{} attribute in the TDS [default kTRUE]. | |
URANIE::DataServer::TDataServer * | _tds |
Int_t | _nS |
Pointer to a TDS. | |
TString | _sInputAttributes |
The string of input attributes (separated by the character ":". | |
TString | _sOutputAttributes |
The string of output attributes (separated by the character ":". | |
TString | _sIntermediateArchitecture |
The intermediate (between the first and last character ",") information. | |
TList * | _listOfInputAttributes |
The list of input Leaf. | |
TList * | _listOfOutputAttributes |
The list of output Leaf. | |
Int_t | _nX |
The number of input attributes. | |
Int_t | _nY |
The number of output attributes. | |
TString | _sFileName |
The name of the file when export the model without extension. | |
TString | _sFunctionName |
The name of the function when export the model. | |
TVectorD | _parameterValues |
The list of parameters. | |
Double_t | _dr2 |
The R2 quality. | |
Bool_t | _bIntercept |
For certain models, add the intercept (Default is TRUE) | |
Detailed Description
Description of the class TKernel.
Member Enumeration Documentation
◆ EKernelType
Constructor & Destructor Documentation
◆ TKernel() [1/2]
URANIE::Modeler::TKernel::TKernel | ( | URANIE::DataServer::TDataServer * | tds, |
TString | architecture, | ||
Option_t * | option = "" |
||
) |
constructor with the TDataServer and the architecture
- Parameters
-
tds (URANIE::DataServer::TDataServer *) the dataserver architecture (TString) The architecture (input and output attributes) of the model separated by the caracter ":" option (Option_t *)[""] The option to pass
Referenced by ClassImp().
◆ TKernel() [2/2]
URANIE::Modeler::TKernel::TKernel | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | varexpinput, | ||
const char * | varexpoutput, | ||
Option_t * | option = "" |
||
) |
Default constructor with input and output attributes.
- Parameters
-
tds (URANIE::DataServer::TDataServer *) the dataserver varexpinput(const char*)[""] The list of input attributes to pass to the function separated by the caracter ":" varexpoutput (const char *)[""] The list of output attributes separated by the caracter ":" option (Option_t *)[""] The option to pass
◆ ~TKernel()
|
virtual |
Default destructor.
Referenced by ClassImp().
Member Function Documentation
◆ computeK()
void URANIE::Modeler::TKernel::computeK | ( | Option_t * | option = "" | ) |
◆ estimate()
void URANIE::Modeler::TKernel::estimate | ( | Option_t * | option = "" | ) |
◆ exportModelCplusplus()
|
virtual |
Export the model in C++ langage in a file.
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (std::ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ exportModelFortran()
|
virtual |
Export the model in Fortran langage in a file.
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (std::ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ exportModelPMML()
|
inlinevirtual |
Export the model in a PMML file (not yet implemented)
- Parameters
-
sourcefile (std::ofstream *) the pointer to the file to export the modeler.
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
Implements URANIE::Modeler::TModeler.
◆ exportModelPython()
|
inlinevirtual |
Export the model in Python langage in a file (not yet implemented)
- Warning
- Don't use this method. Use the main method TModeler::exportFunction.
- Parameters
-
sourcefile (std::ofstream *) the pointer to the file to export the modeler.
Implements URANIE::Modeler::TModeler.
◆ fillData()
void URANIE::Modeler::TKernel::fillData | ( | ) |
Fill the matrix of Inputs attributes and the vector of output attribute (nY=1)
- Todo:
- To deplace in the TModeler Class because it is the same for TLinearRegression.
Referenced by ClassImp().
◆ getKernel()
|
inline |
Return the type of Y distribution.
References _nKernelType.
◆ getLambda()
|
inline |
Get the Lambda.
References _dLambda.
◆ getMatrixK()
|
inline |
◆ getParameter1()
|
inline |
Return First Parameter.
References _dParameter1.
◆ getParameter2()
|
inline |
Return Second Parameter.
References _dParameter2.
◆ printLog()
|
virtual |
Reimplemented from URANIE::Modeler::TModeler.
Referenced by ClassImp().
◆ setKernel() [1/4]
void URANIE::Modeler::TKernel::setKernel | ( | EKernelType | nKernel | ) |
Set the type of kernel without parameter.
- Parameters
-
nKernel (EKernelType) The type of kernel
Referenced by ClassImp(), URANIE::Modeler::TkPCA::setKernel(), URANIE::Modeler::TkPCA::setKernel(), URANIE::Modeler::TkPCA::setKernel(), and URANIE::Modeler::TkPCA::setKernel().
◆ setKernel() [2/4]
void URANIE::Modeler::TKernel::setKernel | ( | EKernelType | nKernel, |
Double_t | dparam1 | ||
) |
- Parameters
-
nKernel (EKernelType) The type of kernel dparam (Double_t) the First and only one parameter
◆ setKernel() [3/4]
void URANIE::Modeler::TKernel::setKernel | ( | EKernelType | nKernel, |
Double_t | dparam1, | ||
Double_t | dparam2 | ||
) |
Set the type of kernel with two parameters.
- Parameters
-
nKernel (EKernelType) The type of kernel dparam1 (Double_t) First parameter dparam2 (Double_t) Second parameter
◆ setKernel() [4/4]
void URANIE::Modeler::TKernel::setKernel | ( | EKernelType | nKernel, |
Double_t | dparam1, | ||
Double_t | dparam2, | ||
Double_t | dparam3 | ||
) |
Set the type of kernel with three parameters.
- Parameters
-
nKernel (EKernelType) The type of kernel dparam1 (Double_t) First parameter dparam2 (Double_t) Second parameter dparam3 (Double_t) Third parameter
◆ setLambda()
|
inline |
Set the Lambda parameter.
References _dLambda.
Member Data Documentation
◆ _dLambda
|
private |
The lambda parameter (default 1.e-6)
Referenced by ClassImp(), getLambda(), and setLambda().
◆ _dParameter1
|
private |
The First Parameter (gamma) [1.0].
Referenced by ClassImp(), and getParameter1().
◆ _dParameter2
|
private |
The Second Parameter (coeff) [0.0].
Referenced by ClassImp(), and getParameter2().
◆ _dParameter3
|
private |
The Third Parameter (degree) [1.0].
Referenced by ClassImp().
◆ _matK
|
private |
The matrix of K (inputs)
Referenced by ClassImp(), and getMatrixK().
◆ _matX
|
private |
The matrix of inputs attributes.
Referenced by ClassImp().
◆ _nKernelType
|
private |
The type of Kernel.
Referenced by ClassImp(), and getKernel().
◆ _nParameter
|
private |
The number of parameter.
Referenced by ClassImp().
◆ _vecY
|
private |
The vector of Y (outputs)
Referenced by ClassImp().