Documentation / Developer's manual
Available modules
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Sampler v4.9.0
|
Description of the class TGaussianSampling. More...
#include <TGaussianSampling.h>
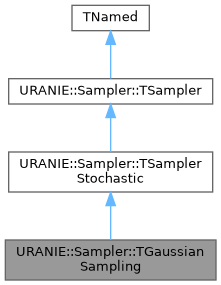
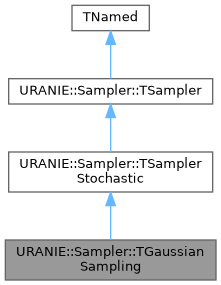
Public Types | |
enum | EType { kLHS , kSRS , kUnknown } |
Public Member Functions | |
Constructor and Destructor | |
TGaussianSampling (URANIE::DataServer::TDataServer *tds, Option_t *option="lhs", Int_t nCalcul=100) | |
Constructor from a TDataServer, the name of the method and the size of the sample. | |
virtual | ~TGaussianSampling () |
Default destructor. | |
State machine | |
For the stochastic methods, two states can be created init and terminate which are executed just before the generation of the sample for the first one, and after the generation for the second one. They can be used for pre and post treatment purpose. | |
virtual void | init () |
The preprocessing step. | |
virtual void | terminate () |
The post-processing step. | |
void | generateSample (Option_t *option="") |
Generates the sample. | |
void | generateConditionalSample (TVectorD vecOf01, Int_t no, Int_t ni) |
void | setIteratorNameNo (TString sNameNo) |
void | setIteratorNameNi (TString sNameNi) |
const char * | getIteratorNameNo () |
const char * | getIteratorNameNi () |
Correlation matrix | |
Methods to specify correlation between attributes | |
void | setUserCorrelation (Int_t indx, Int_t indy, double value) |
Defines a correlation between two attributes given by their indexes. | |
void | setUserVarianceCovarianceMatrix (Int_t indx, Int_t indy, double value) |
void | setUserCorrelation (TString xname, TString yname, double value) |
Defines a correlation between two attributes given by their names. | |
void | setUserCorrelation (URANIE::DataServer::TAttribute *x, URANIE::DataServer::TAttribute *y, Double_t value) |
Defines a correlation between two attributes. | |
void | setFixedSumCovariance (TString method="simple") |
Defines a special covariance matrix. | |
TMatrixD | getCorrelationMatrix () |
Retrieve the correlation matrix. | |
TMatrixD | getCovarianceMatrix () |
Retrieve the covariance matrix. | |
Printing Log | |
virtual void | printLog (Option_t *option="") |
Prints the log. | |
![]() | |
TSamplerStochastic (URANIE::DataServer::TDataServer *tds, Option_t *option, Int_t nCalcul) | |
Constructor with a dataserver. | |
virtual | ~TSamplerStochastic () |
Default destructor. | |
void | setSeed (Int_t ind=0) |
Init the seed. | |
Int_t | getSeed () |
Returns the seed value. | |
![]() | |
TSampler (URANIE::DataServer::TDataServer *tds, Option_t *option, Int_t nCalcul) | |
Constructor with a TDataServer, the options and the size of the sample. | |
virtual | ~TSampler () |
Default destructor. | |
Int_t | GetID () |
Returns the ID of the class. | |
void | setMethodName (TString str) |
Sets the method name in a global variable. | |
TString | getMethodName () |
Gets the method name. | |
virtual URANIE::DataServer::TDataServer * | getTDS () |
Return the TDS filling by the sampling algorithm. | |
void | parseOption (Option_t *option) |
Parse the option. | |
virtual void | createListOfAttributes () |
Creates the List of attributes to simulate. | |
virtual void | createTuple () |
Creates the TDSNtupleD of data with only the TStochasticAttributes. | |
URANIE::DataServer::TDSNtupleD * | getTuple () |
Returns the TDSNtupleD of data. | |
virtual void | fillOtherAttributes () |
Fills the TDSNtupleD of data with other TFormulaAttributes. | |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
Public Attributes | |
TMatrixD | _corrmatrix |
TMatrixD | _covmatrix |
The correlation matrix. | |
EType | _ntype |
The covariance matrix. | |
![]() | |
Int_t | _nS |
The size of the sample. | |
Int_t | _nX |
The size of attributes to sample. | |
URANIE::DataServer::TDSNtupleD * | _ntsample |
the tntuple of data | |
TString | _sMethod |
The title of the sampler method. | |
Bool_t | _blog |
Log Printing. | |
Bool_t | _bupdateFile |
Update the back up file when generating the attributeformula if there is some. | |
URANIE::DataServer::TDataServer * | _tds |
Pointer to a TDS. | |
TList * | _lstOfAttributesToSample |
The list of Stochastic Attributes to sample. | |
Private Member Functions | |
void | splitMatrixVarCoVar (TMatrixD matVarCovar, TVectorD vecOfUnit, TMatrixD &matVarCovar0, TMatrixD &matVarCovar1, TMatrixD &matVarCovar01) |
The type of sampling (SRS, LHS) | |
void | generatenStandardPoints (TMatrixD &matPoints) |
Private Attributes | |
TString | _sIteratorNameNo |
The specific iterator attribute for NO integer. | |
TString | _sIteratorNameNi |
The specific iterator attribute for NI integer. | |
Additional Inherited Members | |
State machine | |
For the stochastic methods, two2 states can be created init and terminate which are executed just before the generation of the sample for the first one, and after the generation for the second one. They can be used for pre and post treatment purpose. | |
![]() | |
Int_t | _nSeed |
Detailed Description
Description of the class TGaussianSampling.
This class provides a data generator for gaussian random variables.
To generate
- the user defines a list of gaussian random variables
. - a covariance matrix
is constructed from the variances of the , and possibly modified by the user (adding covariances) - we apply a Cholesky decomposition on the matrix
, such that , where is an upper triangular matrix. - we build a data matrix
of random values, generated from gaussian random variables , with mean 0 and variance 1 - we apply the formula
, where is a matrix containing the means of the variables .
The output matrix
Member Enumeration Documentation
◆ EType
Constructor & Destructor Documentation
◆ TGaussianSampling()
URANIE::Sampler::TGaussianSampling::TGaussianSampling | ( | URANIE::DataServer::TDataServer * | tds, |
Option_t * | option = "lhs" , |
||
Int_t | nCalcul = 100 |
||
) |
Constructor from a TDataServer, the name of the method and the size of the sample.
- Parameters
-
tds (TDataServer *) the pointer of the TDataServer. It must contains objects inheriting from TStochasticAttribute option (Option_t *) Method of sampling. It can be "lhs" (Latin Hypercube Sampling) or "srs" (Simple Random Sampling) (Default = "lhs"). nCalcul (Int_t) number of values to generate for each attribute (Default = 100)
Referenced by ClassImp().
◆ ~TGaussianSampling()
|
virtual |
Default destructor.
Referenced by ClassImp().
Member Function Documentation
◆ generateConditionalSample()
void URANIE::Sampler::TGaussianSampling::generateConditionalSample | ( | TVectorD | vecOf01, |
Int_t | no, | ||
Int_t | ni | ||
) |
Referenced by ClassImp().
◆ generatenStandardPoints()
|
private |
Referenced by ClassImp().
◆ generateSample()
|
virtual |
◆ getCorrelationMatrix()
|
inline |
Retrieve the correlation matrix.
References _corrmatrix.
◆ getCovarianceMatrix()
|
inline |
Retrieve the covariance matrix.
References _covmatrix.
◆ getIteratorNameNi()
|
inline |
References _sIteratorNameNi.
◆ getIteratorNameNo()
|
inline |
References _sIteratorNameNo.
◆ init()
|
virtual |
The preprocessing step.
Reimplemented from URANIE::Sampler::TSamplerStochastic.
Referenced by ClassImp().
◆ printLog()
|
virtual |
◆ setFixedSumCovariance()
void URANIE::Sampler::TGaussianSampling::setFixedSumCovariance | ( | TString | method = "simple" | ) |
Defines a special covariance matrix.
The covariance matrix is defined such that the sum of the generated values is always equal to the sum of the random variables' means.
- Warning
- THIS METHOD IS EXPERIMENTAL. Its advantage is to preserve the "gaussian nature" of the generated data. This might be important when working on a small number of variables.
- Parameters
-
method (TString) method to compute the covariance matrix. Two are available: the "simple" method is very simple to implement but may be loose on standard deviations reconstruction. The "exact" method is more tricky but should preserve the standard deviations.
(Default = "simple")
Referenced by ClassImp().
◆ setIteratorNameNi()
|
inline |
References _sIteratorNameNi.
◆ setIteratorNameNo()
|
inline |
References _sIteratorNameNo.
◆ setUserCorrelation() [1/3]
void URANIE::Sampler::TGaussianSampling::setUserCorrelation | ( | Int_t | indx, |
Int_t | indy, | ||
double | value | ||
) |
Defines a correlation between two attributes given by their indexes.
- Warning
- The indexes' range is from 0 to n-1.
- Parameters
-
indx (Int_t) the index of the first attribute indy (Int_t) the index of the second attribute value (Double_t) the correlation factor between the two attributes
Referenced by ClassImp().
◆ setUserCorrelation() [2/3]
void URANIE::Sampler::TGaussianSampling::setUserCorrelation | ( | TString | xname, |
TString | yname, | ||
double | value | ||
) |
Defines a correlation between two attributes given by their names.
- Parameters
-
xname (TString) the first attribute yname (TString) the second attribute value (Double_t) the correlation factor between x and y
◆ setUserCorrelation() [3/3]
void URANIE::Sampler::TGaussianSampling::setUserCorrelation | ( | URANIE::DataServer::TAttribute * | x, |
URANIE::DataServer::TAttribute * | y, | ||
Double_t | value | ||
) |
Defines a correlation between two attributes.
- Parameters
-
x (URANIE::DataServer::TAttribute *) the first attribute y (URANIE::DataServer::TAttribute *) the second attribute value (Double_t) the correlation factor between x and y
◆ setUserVarianceCovarianceMatrix()
void URANIE::Sampler::TGaussianSampling::setUserVarianceCovarianceMatrix | ( | Int_t | indx, |
Int_t | indy, | ||
double | value | ||
) |
Referenced by ClassImp().
◆ splitMatrixVarCoVar()
|
private |
The type of sampling (SRS, LHS)
Referenced by ClassImp().
◆ terminate()
|
virtual |
The post-processing step.
Reimplemented from URANIE::Sampler::TSamplerStochastic.
Referenced by ClassImp().
Member Data Documentation
◆ _corrmatrix
TMatrixD URANIE::Sampler::TGaussianSampling::_corrmatrix |
Referenced by ClassImp(), and getCorrelationMatrix().
◆ _covmatrix
TMatrixD URANIE::Sampler::TGaussianSampling::_covmatrix |
The correlation matrix.
Referenced by ClassImp(), and getCovarianceMatrix().
◆ _ntype
EType URANIE::Sampler::TGaussianSampling::_ntype |
The covariance matrix.
Referenced by ClassImp().
◆ _sIteratorNameNi
|
private |
The specific iterator attribute for NI integer.
Referenced by ClassImp(), getIteratorNameNi(), and setIteratorNameNi().
◆ _sIteratorNameNo
|
private |
The specific iterator attribute for NO integer.
Referenced by ClassImp(), getIteratorNameNo(), and setIteratorNameNo().