Documentation / Developer's manual
Available modules
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Uncertainty Modeler v4.9.0
|
#include <TCirce.h>
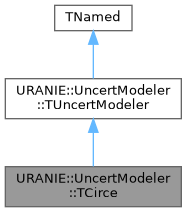
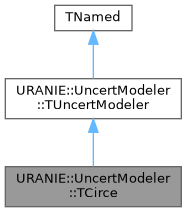
Public Types | |
enum | ECirceMethod { kBiais , kBiaisBloc , kBiaisBlocCInitAlea , kBiaisBlocBootstrap , kUnknown } |
Public Member Functions | |
Constructor and Destructor | |
TCirce (URANIE::DataServer::TDataServer *tds, const char *systar, const char *syhat, const char *ssensi, const char *sexpuncert="", Option_t *option="") | |
Default constructor. | |
virtual | ~TCirce () |
Default destructor. | |
Setting and Getting attributs | |
Treat the case where you have a Circe Input File | |
void | setTolerance (Double_t dtol) |
Set the tolerance parameter. | |
Double_t | getTolerance () |
Get the Tolerance parameter. | |
void | setNCMatrix (Int_t n) |
Set the number of random matrix C to generate. | |
Int_t | getNCMatrix () |
Get the number of random matrix C to generate. | |
Int_t | getNTDS () |
Get the number of TDS. | |
Double_t | getLikelihood () |
Get the Likelihood for the optimal parameters. | |
Int_t | getNPatterns () |
Get the number of patterns. | |
Int_t | getNParameters () |
Get the number of parameters. | |
TMatrixD | getMatrixVarianceMu () |
Get the matrix of variance of mu. | |
TMatrixD | getMatrixVarianceSigma () |
Get the matrix of variance of sigma. | |
vector B and matrix C Initial and final values | |
void | setCMatrixInitial (TMatrixD mat) |
Set The initial Matrix C. | |
TMatrixD | getCMatrixInitial () |
Get the initial Matrix C. | |
TMatrixD | getCMatrix () |
Get the final matrix C of coriance (Circe) | |
void | setBVectorInitial (TVectorD vec) |
Set the initial vector B. | |
TVectorD | getBVectorInitial () |
Get the initial vector B. | |
TVectorD | getBVector () |
Get the final vector of biais (Circe) | |
Methods for the algorithm | |
void | init (Option_t *option="") |
The init method. | |
void | estimate (Option_t *option="") |
the estimate procedure | |
void | terminate (Option_t *option="") |
the terminate method | |
void | printResults (Option_t *option="") |
Print the results. | |
void | addData (URANIE::DataServer::TDataServer *tds, const char *systar, const char *syhat, const char *ssensi, const char *sexpuncert="") |
Add data from a new TDS. | |
Other methods for Direct implementation | |
void | setInputFile (const char *sfile) |
Set the Circe Input File. | |
const char * | getInputFile () |
Get the Circe Input File. | |
void | readInputFile (const char *sfile="", ECirceMethod nCirceMethod=kBiaisBloc) |
Set and Read the Circe Input File. | |
Internal methods | |
void | addBloc (URANIE::DataServer::TDataServer *tds, const char *sbloc, const char *sname="") |
The addBloc method. | |
TMatrixD | initCMatrix (Int_t nrows, Double_t dMulti) |
Generate a ramdom finite positive matrix C. | |
The Seed | |
void | setSeed (Int_t ind=0) |
Init the seed. | |
Int_t | getSeed () |
Returns the seed value. | |
Printing Log | |
virtual void | printLog (Option_t *option="") |
Print the log of the class. | |
![]() | |
TUncertModeler (URANIE::DataServer::TDataServer *tds, const char *stest, const char *varexpinput, Option_t *option="") | |
Default constructor. | |
virtual | ~TUncertModeler () |
Default constructor. | |
Int_t | getID () |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
Protected Attributes | |
Int_t | _nSeed |
the Seed of the random generator | |
Double_t | _dTol |
The tolerance to test the convergence (default 1e-5) | |
Double_t | _dLikelihood |
The Likelihood for the optimal parameter. | |
TVectorD | _vecBiais |
The final vector of biais. | |
TMatrixD | _matC |
The final matrix of Covariance. | |
TMatrixD | _matCorrelation |
The final matrix of correlation. | |
Int_t | _nPatterns |
The number of patterns. | |
Int_t | _nParameters |
The number of parameters. | |
TString | _sCirceInputFile |
The input file of Circe. | |
ECirceMethod | _nCirceMethod |
the Circe method from (kBiais, kBiaisBloc, kBiaisBlocCInitAlea, kBiaisBlocBootstrap) | |
Int_t | _nInitCAlea |
the number of Matrix C alea (default 1) | |
TList * | _listOfTDS |
The list of TDS which contain data. | |
TList * | _listOfInformations |
The list of information for Circe. | |
TList * | _listOfBloc |
The list of bloc (default NULL : when only one is as blias.f) | |
TList * | _listOfSensitivity |
The list of sensitivity parameter. | |
TVectorD | _vecDiff |
The vector Y = Texp - Ycode. | |
TVectorD | _vecYStarVariance |
The vector of experimental variance. | |
TMatrixD | _matSensibility |
The matrix of sensibility. | |
TMatrixD | _matInitialC |
The initial matrix of C. | |
TVectorD | _vecInitialB |
The initial vector B. | |
TMatrixD | _matVarianceMu |
The matrix of variance of mu computed Confidence Interval by the Fisher Matrix. | |
TMatrixD | _matVarianceSigma |
The matrix of variance of sigma computed Confidence Interval by the Fisher Matrix. | |
![]() | |
Char_t | _sType [12] |
Char_t | _sMethod [12] |
Float_t | _pValue |
Additional Inherited Members | |
![]() | |
URANIE::DataServer::TDataServer * | _tds |
Pointer to a TDS. | |
Bool_t | _blog |
Boolean to edit the log. | |
Member Enumeration Documentation
◆ ECirceMethod
Constructor & Destructor Documentation
◆ TCirce()
URANIE::UncertModeler::TCirce::TCirce | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | systar, | ||
const char * | syhat, | ||
const char * | ssensi, | ||
const char * | sexpuncert = "" , |
||
Option_t * | option = "" |
||
) |
◆ ~TCirce()
|
virtual |
Default destructor.
References _listOfBloc, _listOfInformations, _listOfSensitivity, and _listOfTDS.
Member Function Documentation
◆ addBloc()
void URANIE::UncertModeler::TCirce::addBloc | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | sbloc, | ||
const char * | sname = "" |
||
) |
The addBloc method.
References URANIE::UncertModeler::TUncertModeler::_blog, _listOfBloc, _listOfSensitivity, and CIRCE_BLOC_NAME.
◆ addData()
void URANIE::UncertModeler::TCirce::addData | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | systar, | ||
const char * | syhat, | ||
const char * | ssensi, | ||
const char * | sexpuncert = "" |
||
) |
Add data from a new TDS.
References URANIE::UncertModeler::TUncertModeler::_blog, _listOfInformations, _listOfSensitivity, _listOfTDS, _nParameters, addBloc(), and CIRCE_RESIDU_ATTRIBUTE.
Referenced by TCirce().
◆ estimate()
void URANIE::UncertModeler::TCirce::estimate | ( | Option_t * | option = "" | ) |
the estimate procedure
References URANIE::UncertModeler::TUncertModeler::_blog, _dLikelihood, _dTol, _listOfSensitivity, _listOfTDS, _matC, _matCorrelation, _matInitialC, _matSensibility, _nInitCAlea, _nPatterns, URANIE::UncertModeler::TUncertModeler::_tds, _vecBiais, _vecDiff, _vecInitialB, _vecYStarVariance, CIRCE_RESIDU_ATTRIBUTE, init(), initCMatrix(), and terminate().
◆ getBVector()
|
inline |
Get the final vector of biais (Circe)
References _vecBiais.
◆ getBVectorInitial()
|
inline |
Get the initial vector B.
References _vecInitialB.
◆ getCMatrix()
|
inline |
Get the final matrix C of coriance (Circe)
References _matC.
◆ getCMatrixInitial()
|
inline |
Get the initial Matrix C.
References _matInitialC.
◆ getInputFile()
|
inline |
Get the Circe Input File.
References _sCirceInputFile.
◆ getLikelihood()
|
inline |
Get the Likelihood for the optimal parameters.
References _dLikelihood.
◆ getMatrixVarianceMu()
|
inline |
Get the matrix of variance of mu.
References _matVarianceMu.
◆ getMatrixVarianceSigma()
|
inline |
Get the matrix of variance of sigma.
References _matVarianceSigma.
◆ getNCMatrix()
|
inline |
Get the number of random matrix C to generate.
References _nInitCAlea.
◆ getNParameters()
|
inline |
Get the number of parameters.
References _nParameters.
◆ getNPatterns()
|
inline |
Get the number of patterns.
References _nPatterns.
◆ getNTDS()
Int_t URANIE::UncertModeler::TCirce::getNTDS | ( | ) |
Get the number of TDS.
References _listOfTDS.
◆ getSeed()
|
inline |
Returns the seed value.
References _nSeed.
◆ getTolerance()
|
inline |
Get the Tolerance parameter.
References _dTol.
◆ init()
void URANIE::UncertModeler::TCirce::init | ( | Option_t * | option = "" | ) |
The init method.
References URANIE::UncertModeler::TUncertModeler::_blog, _listOfInformations, _listOfSensitivity, _listOfTDS, _matSensibility, _nPatterns, _vecDiff, _vecYStarVariance, and addBloc().
Referenced by estimate().
◆ initCMatrix()
TMatrixD URANIE::UncertModeler::TCirce::initCMatrix | ( | Int_t | nrows, |
Double_t | dMulti | ||
) |
Generate a ramdom finite positive matrix C.
References URANIE::UncertModeler::TUncertModeler::_blog.
Referenced by estimate().
◆ printLog()
|
virtual |
Print the log of the class.
Reimplemented from URANIE::UncertModeler::TUncertModeler.
References _dLikelihood, _dTol, _listOfBloc, _listOfSensitivity, _matSensibility, _nCirceMethod, _nParameters, _nPatterns, _nSeed, _sCirceInputFile, _vecDiff, _vecYStarVariance, CIRCE_DIFF_ATTRIBUTE, kBiais, kBiaisBloc, kBiaisBlocBootstrap, kBiaisBlocCInitAlea, and URANIE::UncertModeler::TUncertModeler::printLog().
◆ printResults()
void URANIE::UncertModeler::TCirce::printResults | ( | Option_t * | option = "" | ) |
Print the results.
References _dLikelihood, _dTol, _listOfSensitivity, _matC, _matVarianceMu, _matVarianceSigma, _nCirceMethod, _nParameters, _nPatterns, _nSeed, _sCirceInputFile, _vecBiais, CIRCE_DIFF_ATTRIBUTE, kBiais, kBiaisBloc, kBiaisBlocBootstrap, and kBiaisBlocCInitAlea.
◆ readInputFile()
void URANIE::UncertModeler::TCirce::readInputFile | ( | const char * | sfile = "" , |
ECirceMethod | nCirceMethod = kBiaisBloc |
||
) |
Set and Read the Circe Input File.
◆ setBVectorInitial()
void URANIE::UncertModeler::TCirce::setBVectorInitial | ( | TVectorD | vec | ) |
Set the initial vector B.
References URANIE::UncertModeler::TUncertModeler::_blog, and _vecInitialB.
◆ setCMatrixInitial()
void URANIE::UncertModeler::TCirce::setCMatrixInitial | ( | TMatrixD | mat | ) |
Set The initial Matrix C.
References URANIE::UncertModeler::TUncertModeler::_blog, and _matInitialC.
◆ setInputFile()
void URANIE::UncertModeler::TCirce::setInputFile | ( | const char * | sfile | ) |
Set the Circe Input File.
References _sCirceInputFile.
◆ setNCMatrix()
|
inline |
Set the number of random matrix C to generate.
References _nInitCAlea.
◆ setSeed()
void URANIE::UncertModeler::TCirce::setSeed | ( | Int_t | ind = 0 | ) |
◆ setTolerance()
void URANIE::UncertModeler::TCirce::setTolerance | ( | Double_t | dtol | ) |
Set the tolerance parameter.
References URANIE::UncertModeler::TUncertModeler::_blog, and _dTol.
◆ terminate()
void URANIE::UncertModeler::TCirce::terminate | ( | Option_t * | option = "" | ) |
the terminate method
References URANIE::UncertModeler::TUncertModeler::_blog, _matC, _matSensibility, _matVarianceMu, _matVarianceSigma, _nParameters, _nPatterns, and _vecYStarVariance.
Referenced by estimate().
Member Data Documentation
◆ _dLikelihood
|
protected |
The Likelihood for the optimal parameter.
Referenced by estimate(), getLikelihood(), printLog(), and printResults().
◆ _dTol
|
protected |
The tolerance to test the convergence (default 1e-5)
Referenced by estimate(), getTolerance(), printLog(), printResults(), and setTolerance().
◆ _listOfBloc
|
protected |
The list of bloc (default NULL : when only one is as blias.f)
Referenced by addBloc(), printLog(), and ~TCirce().
◆ _listOfInformations
|
protected |
◆ _listOfSensitivity
|
protected |
The list of sensitivity parameter.
Referenced by addBloc(), addData(), estimate(), init(), printLog(), printResults(), and ~TCirce().
◆ _listOfTDS
|
protected |
The list of TDS which contain data.
Referenced by addData(), estimate(), getNTDS(), init(), and ~TCirce().
◆ _matC
|
protected |
The final matrix of Covariance.
Referenced by estimate(), getCMatrix(), printResults(), and terminate().
◆ _matCorrelation
|
protected |
The final matrix of correlation.
Referenced by estimate().
◆ _matInitialC
|
protected |
The initial matrix of C.
Referenced by estimate(), getCMatrixInitial(), and setCMatrixInitial().
◆ _matSensibility
|
protected |
The matrix of sensibility.
Referenced by estimate(), init(), printLog(), and terminate().
◆ _matVarianceMu
|
protected |
The matrix of variance of mu computed Confidence Interval by the Fisher Matrix.
Referenced by getMatrixVarianceMu(), printResults(), and terminate().
◆ _matVarianceSigma
|
protected |
The matrix of variance of sigma computed Confidence Interval by the Fisher Matrix.
Referenced by getMatrixVarianceSigma(), printResults(), and terminate().
◆ _nCirceMethod
|
protected |
the Circe method from (kBiais, kBiaisBloc, kBiaisBlocCInitAlea, kBiaisBlocBootstrap)
Referenced by printLog(), and printResults().
◆ _nInitCAlea
|
protected |
the number of Matrix C alea (default 1)
Referenced by estimate(), getNCMatrix(), and setNCMatrix().
◆ _nParameters
|
protected |
The number of parameters.
Referenced by addData(), getNParameters(), printLog(), printResults(), and terminate().
◆ _nPatterns
|
protected |
The number of patterns.
Referenced by estimate(), getNPatterns(), init(), printLog(), printResults(), and terminate().
◆ _nSeed
|
protected |
the Seed of the random generator
Referenced by getSeed(), printLog(), printResults(), and setSeed().
◆ _sCirceInputFile
|
protected |
The input file of Circe.
Referenced by getInputFile(), printLog(), printResults(), and setInputFile().
◆ _vecBiais
|
protected |
The final vector of biais.
Referenced by estimate(), getBVector(), and printResults().
◆ _vecDiff
|
protected |
The vector Y = Texp - Ycode.
Referenced by estimate(), init(), and printLog().
◆ _vecInitialB
|
protected |
The initial vector B.
Referenced by estimate(), getBVectorInitial(), and setBVectorInitial().
◆ _vecYStarVariance
|
protected |
The vector of experimental variance.
Referenced by estimate(), init(), printLog(), and terminate().