Documentation / Manuel développeur
Modules disponibles
Calibration,  DataServer,  Launcher,  MetaModelOptim,  Modeler,  Optimizer,  ReLauncher,  Reliability,  ReOptimizer,  Sampler,  Sensitivity,  UncertModeler,  XmlProblem,  ![]() |
Uranie / Optimizer v4.9.0
|
Description of the class TOptimizer. More...
#include <TOptimizer.h>
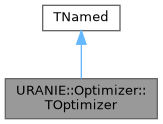
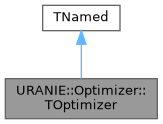
Public Types | |
enum | EOptimType { kMinimizeCode , kMinimizeFunction , kSumOfSquare } |
enum | EOptimMethod { kMigrad , kSimplex , kCombined , kScan } |
Public Member Functions | |
Constructor and Destructor | |
TOptimizer (URANIE::DataServer::TDataServer *tds, URANIE::Launcher::TCode *code) | |
Constructor with a dataserver. | |
TOptimizer (URANIE::DataServer::TDataServer *tds, void(*fcn)(Double_t *, Double_t *), TString sinput, TString soutput) | |
Constructor by a TDataServer, a function and list of input and output (optional) | |
TOptimizer (URANIE::DataServer::TDataServer *tds, const char *fcn, TString sinput="", TString soutput="") | |
Constructor by a TDataServer, a function name and list of input and output (optional) | |
virtual | ~TOptimizer () |
Default destructor. | |
Set/Get parameters | |
void | setTolerance (Double_t dtol) |
Set the tolerance parameter. | |
Double_t | getTolerance () |
Get the tolerance parameter. | |
void | setMaxIterations (Int_t nmax) |
Set the maximum number of iterations. | |
Int_t | getMaxIterations () |
Get the maximum number of iterations. | |
void | setMaxFunctionCalls (Int_t nmax) |
Set the maximum number of evaluations. | |
Int_t | getMaxFunctionCalls () |
Get the maximum numbers of evaluations. | |
Set/Get the method of Optimization | |
These classe is a wrapper with the TMinuit library interfaced in ROOT which contains several algorithm of optimization :
| |
void | setMethod (EOptimMethod method=kMigrad) |
Select the algorithm of optimization. | |
EOptimMethod | getMethod () |
Return the algorithm of optimization. | |
EOptimType | getOptimType () |
Return the type of optimization. | |
void | setPrintLevel (int level) |
Set the print Level of Debug between ![]() | |
int | getPrintLevel () const |
Get the print Level of Debug between ![]() | |
Manipulate the code | |
virtual void | init (Option_t *option="") |
Init phase of the code. | |
virtual void | optimize (Option_t *option="") |
Runs phase of the code (TMinuit2) | |
virtual void | terminate (Option_t *option="") |
Terminates phase of the code. | |
virtual void | clean (Option_t *option="") |
Cleans the working directory of the code. | |
Manipulate the Objectives | |
In the case of calibration of code, we can define one or several "Objectives" to specify the criteria to minimize. The criteria to minimize is a sum of weighted objectives:
By default, all added objectives are "active" and the weight | |
void | addObjective (TString name, URANIE::DataServer::TDataServer *tds, TString ystar, URANIE::Launcher::TOutputFile *outfile, TString yhat, Double_t weight=1.0) |
Add the objective in the TOptimizer object : Sum of square of the error. | |
void | addObjective (TString name, URANIE::DataServer::TDataServer *tds, TString ystar, TString sigma, URANIE::Launcher::TOutputFile *outfile, TString yhat, Double_t alpha=1.0) |
Add the objective in the TOptimizer object : Sum of square of the weighted error. | |
void | unactiveObjective (TString name) |
Unactive the objective given by these name. | |
void | activeObjective (TString name) |
Active the objective given by these name. | |
void | invertObjectives () |
Invert the state of all the objectives. | |
vector< URANIE::Optimizer::TObjective * > | getObjectives () |
get the vector containing the objectives | |
void | addOutputVariable (TString soutput) |
Add input and output variables. | |
void | selectCost (TString scost) |
Select an cost in the Output string. | |
TString | getSelectedCost () |
Get the selected Select an cost in the Output string. | |
Manipulate the parameters | |
At any time, we can change the state of the parameters; it can be fix to the default value or it can be variable in these range. | |
void | fixParameter (TString name) |
Fix the parameter given by these name to the default value. | |
void | unfixParameter (TString name) |
Unfix the parameter given by these name. | |
void | invertParameters () |
Invert the state of all the parameters. | |
Printing Log | |
void | printState (Option_t *option="") |
Print the state of the algorithm of optimization. | |
void | setLog () |
void | unsetLog () |
void | changeLog () |
Bool_t | getLog () |
virtual void | printLog (Option_t *option="") |
Private Attributes | |
TString | _sdirectory |
Current directory. | |
Bool_t | _bsave |
Saves all the computation files in each directory. | |
Bool_t | _bclean |
Cleans the Working Directory before running the code. | |
Bool_t | _blog |
Boolean to edit the log. | |
Int_t | _nPrintLevel |
The level of Debg between ![]() | |
URANIE::DataServer::TDataServer * | _tds |
URANIE::Launcher::TCode * | _code |
Pointer vers un TDS. | |
Int_t | _nMaxIterations |
Pointer vers un TCode. | |
Int_t | _nMaxFunctionCalls |
The max number to call the code/function. | |
Double_t | _dTolerance |
The tolerance parameter. | |
vector< TObjective * > | _objectives |
vector of objectives | |
TMethodCall * | _fMethodCall |
Pointer to MethodCall in case of interpreted function. | |
void(* | _fMethod )(Double_t *, Double_t *) |
TString | _sFunctionName |
A pointer for the analytical function. | |
EOptimType | _optimProblem |
Function name. | |
EOptimMethod | _optimMethod |
The method of optimisation. | |
TString | _sInput |
The string of input attributes. | |
TString | _sOutput |
The string of output attributes (the number must be equal to 1) | |
TString | _sCost |
The name od the selected cost (the number must be equal to 1) | |
Detailed Description
Description of the class TOptimizer.
To be written by the developper.
Member Enumeration Documentation
◆ EOptimMethod
◆ EOptimType
Constructor & Destructor Documentation
◆ TOptimizer() [1/3]
URANIE::Optimizer::TOptimizer::TOptimizer | ( | URANIE::DataServer::TDataServer * | tds, |
URANIE::Launcher::TCode * | code | ||
) |
Constructor with a dataserver.
Referenced by ClassImp().
◆ TOptimizer() [2/3]
URANIE::Optimizer::TOptimizer::TOptimizer | ( | URANIE::DataServer::TDataServer * | tds, |
void(*)(Double_t *, Double_t *) | fcn, | ||
TString | sinput, | ||
TString | soutput | ||
) |
Constructor by a TDataServer, a function and list of input and output (optional)
◆ TOptimizer() [3/3]
URANIE::Optimizer::TOptimizer::TOptimizer | ( | URANIE::DataServer::TDataServer * | tds, |
const char * | fcn, | ||
TString | sinput = "" , |
||
TString | soutput = "" |
||
) |
Constructor by a TDataServer, a function name and list of input and output (optional)
◆ ~TOptimizer()
|
virtual |
Default destructor.
Referenced by ClassImp().
Member Function Documentation
◆ activeObjective()
void URANIE::Optimizer::TOptimizer::activeObjective | ( | TString | name | ) |
Active the objective given by these name.
Referenced by ClassImp().
◆ addObjective() [1/2]
void URANIE::Optimizer::TOptimizer::addObjective | ( | TString | name, |
URANIE::DataServer::TDataServer * | tds, | ||
TString | ystar, | ||
TString | sigma, | ||
URANIE::Launcher::TOutputFile * | outfile, | ||
TString | yhat, | ||
Double_t | alpha = 1.0 |
||
) |
Add the objective in the TOptimizer object : Sum of square of the weighted error.
The objective is computed with formula
- Parameters
-
name (TString) the name of the TObjective tds (TDataServer *) The pointer of the TDataServer object which contains the attribute ystar (TString) the name of the attributesigma (TString) the name of the weight attributeoutfile (TOutputFile *) The pointer of the TOutputFile object which contains the attribute yhat (TString) the name of the attributealpha (Double_t) The weight of the objective [1.0]
◆ addObjective() [2/2]
void URANIE::Optimizer::TOptimizer::addObjective | ( | TString | name, |
URANIE::DataServer::TDataServer * | tds, | ||
TString | ystar, | ||
URANIE::Launcher::TOutputFile * | outfile, | ||
TString | yhat, | ||
Double_t | weight = 1.0 |
||
) |
Add the objective in the TOptimizer object : Sum of square of the error.
The objective is computed with formula
- Parameters
-
name (TString) the name of the TObjective tds (TDataServer *) The pointer of the TDataServer object which contains the attribute ystar (TString) the name of the attributeoutfile (TOutputFile *) The pointer of the TOutputFile object which contains the attribute yhat (TString) the name of the attributeweight (Double_t) The weight of the objective [1.0]
Referenced by ClassImp().
◆ addOutputVariable()
void URANIE::Optimizer::TOptimizer::addOutputVariable | ( | TString | soutput | ) |
Add input and output variables.
Add output attributes defined from other attributes
- Parameters
-
soutput (TString): list of output variable to be created
Referenced by ClassImp().
◆ changeLog()
|
inline |
References _blog.
◆ clean()
|
virtual |
Cleans the working directory of the code.
Referenced by ClassImp().
◆ fixParameter()
void URANIE::Optimizer::TOptimizer::fixParameter | ( | TString | name | ) |
Fix the parameter given by these name to the default value.
Referenced by ClassImp().
◆ getLog()
|
inline |
References _blog.
◆ getMaxFunctionCalls()
|
inline |
Get the maximum numbers of evaluations.
References _nMaxFunctionCalls.
◆ getMaxIterations()
|
inline |
Get the maximum number of iterations.
References _nMaxIterations.
◆ getMethod()
|
inline |
Return the algorithm of optimization.
References _optimMethod.
◆ getObjectives()
|
inline |
get the vector containing the objectives
References _objectives.
◆ getOptimType()
|
inline |
Return the type of optimization.
References _optimProblem.
◆ getPrintLevel()
|
inline |
Get the print Level of Debug between
References _nPrintLevel.
◆ getSelectedCost()
|
inline |
Get the selected Select an cost in the Output string.
References _sCost.
◆ getTolerance()
|
inline |
Get the tolerance parameter.
References _dTolerance.
◆ init()
|
virtual |
Init phase of the code.
Referenced by ClassImp().
◆ invertObjectives()
void URANIE::Optimizer::TOptimizer::invertObjectives | ( | ) |
Invert the state of all the objectives.
Referenced by ClassImp().
◆ invertParameters()
void URANIE::Optimizer::TOptimizer::invertParameters | ( | ) |
Invert the state of all the parameters.
Then, fixed parameters became "variable" attribute and vice versa.
Referenced by ClassImp().
◆ optimize()
|
virtual |
Runs phase of the code (TMinuit2)
Referenced by ClassImp().
◆ printLog()
|
virtual |
Referenced by ClassImp().
◆ printState()
void URANIE::Optimizer::TOptimizer::printState | ( | Option_t * | option = "" | ) |
Print the state of the algorithm of optimization.
Referenced by ClassImp().
◆ selectCost()
void URANIE::Optimizer::TOptimizer::selectCost | ( | TString | scost | ) |
Select an cost in the Output string.
Referenced by ClassImp().
◆ setLog()
|
inline |
References _blog.
◆ setMaxFunctionCalls()
|
inline |
Set the maximum number of evaluations.
References _nMaxFunctionCalls.
◆ setMaxIterations()
|
inline |
Set the maximum number of iterations.
References _nMaxIterations.
◆ setMethod()
|
inline |
Select the algorithm of optimization.
References _optimMethod.
◆ setPrintLevel()
|
inline |
Set the print Level of Debug between
References _nPrintLevel.
◆ setTolerance()
|
inline |
Set the tolerance parameter.
References _dTolerance.
◆ terminate()
|
virtual |
Terminates phase of the code.
Referenced by ClassImp().
◆ unactiveObjective()
void URANIE::Optimizer::TOptimizer::unactiveObjective | ( | TString | name | ) |
Unactive the objective given by these name.
Referenced by ClassImp().
◆ unfixParameter()
void URANIE::Optimizer::TOptimizer::unfixParameter | ( | TString | name | ) |
Unfix the parameter given by these name.
These parameter became a "variable" attribute
Referenced by ClassImp().
◆ unsetLog()
|
inline |
References _blog.
Member Data Documentation
◆ _bclean
|
private |
Cleans the Working Directory before running the code.
Referenced by ClassImp().
◆ _blog
|
private |
Boolean to edit the log.
Referenced by changeLog(), ClassImp(), getLog(), setLog(), and unsetLog().
◆ _bsave
|
private |
Saves all the computation files in each directory.
Referenced by ClassImp().
◆ _code
|
private |
Pointer vers un TDS.
Referenced by ClassImp().
◆ _dTolerance
|
private |
The tolerance parameter.
Referenced by ClassImp(), getTolerance(), and setTolerance().
◆ _fMethod
|
private |
Referenced by ClassImp().
◆ _fMethodCall
|
private |
Pointer to MethodCall in case of interpreted function.
◆ _nMaxFunctionCalls
|
private |
The max number to call the code/function.
Referenced by ClassImp(), getMaxFunctionCalls(), and setMaxFunctionCalls().
◆ _nMaxIterations
|
private |
Pointer vers un TCode.
The max number of iterations
Referenced by ClassImp(), getMaxIterations(), and setMaxIterations().
◆ _nPrintLevel
|
private |
The level of Debg between
Referenced by ClassImp(), getPrintLevel(), and setPrintLevel().
◆ _objectives
|
private |
vector of objectives
Referenced by ClassImp(), and getObjectives().
◆ _optimMethod
|
private |
The method of optimisation.
Referenced by ClassImp(), getMethod(), and setMethod().
◆ _optimProblem
|
private |
◆ _sCost
|
private |
The name od the selected cost (the number must be equal to 1)
Referenced by ClassImp(), and getSelectedCost().
◆ _sdirectory
|
private |
Current directory.
Referenced by ClassImp().
◆ _sFunctionName
|
private |
A pointer for the analytical function.
Referenced by ClassImp().
◆ _sInput
|
private |
The string of input attributes.
Referenced by ClassImp().
◆ _sOutput
|
private |
The string of output attributes (the number must be equal to 1)
Referenced by ClassImp().
◆ _tds
|
private |
Referenced by ClassImp().